Secure Token Generation: Zero-Trust Key Management for iOS
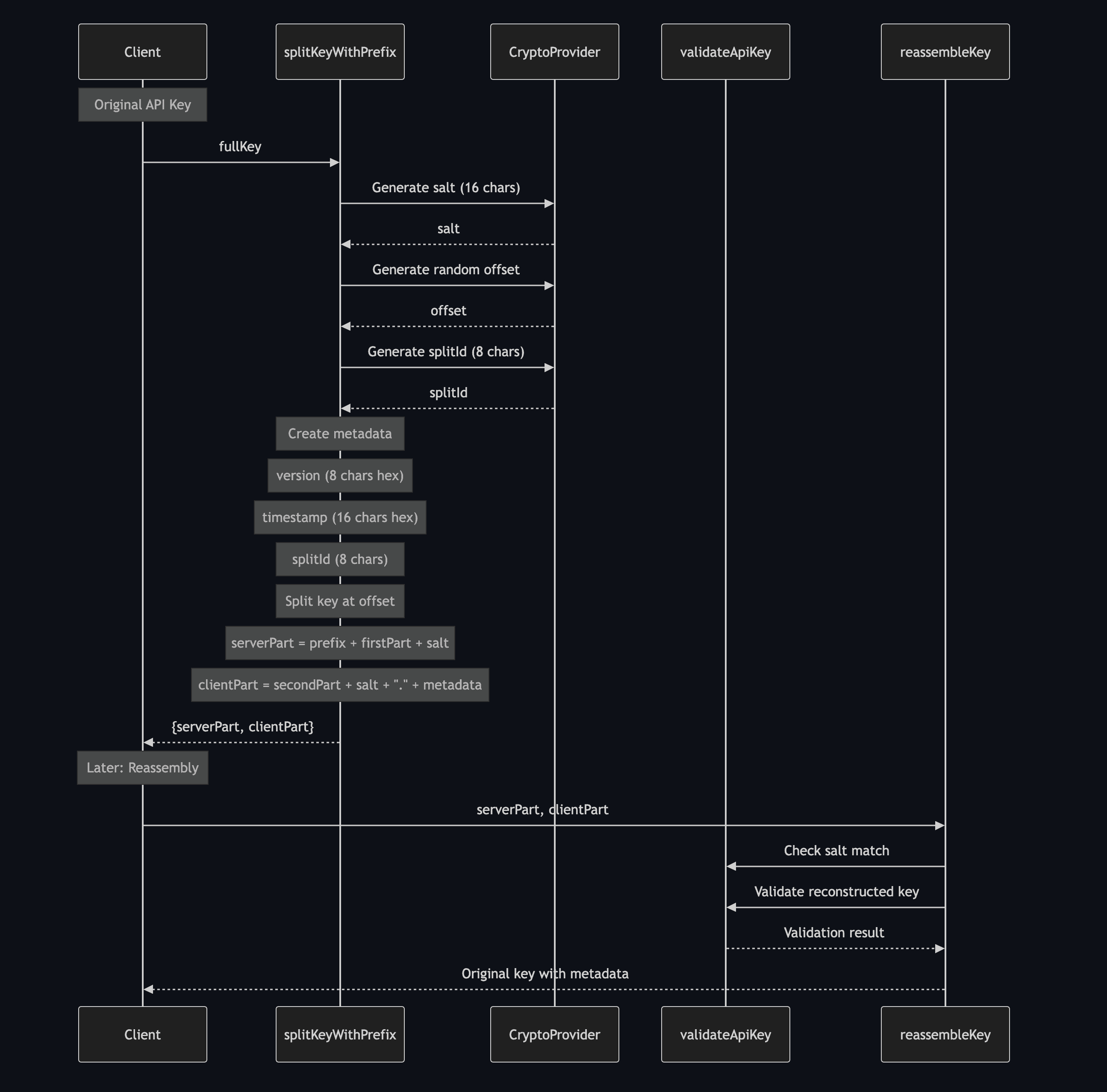
At Proxed, we've developed a unique approach to API key security that combines split-key architecture with Apple's DeviceCheck system. This ensures your API keys remain secure even if any single component is compromised.
#The Challenge
Traditional API key management approaches have several vulnerabilities:
- Complete keys stored on servers create single points of failure
- Keys exposed in mobile apps can be extracted through reverse engineering
- Difficult to track and control key usage effectively
- No way to verify legitimate device usage
#Our Solution: Multi-Layer Security
We've built a system that combines three security layers:
- Split-Key Architecture: Keys are split between server and client
- Apple DeviceCheck: Cryptographic verification of legitimate iOS devices
- Metadata Tracking: Version control and usage monitoring
This approach ensures:
- No complete key exists in any single location
- Only genuine iOS devices can make requests
- Every key usage is tracked and auditable
#Token Structure
Our token structure is designed for security and traceability:
#How It Works
The process combines client-side key splitting with device verification:
-
Key Splitting (Client-Side):
- Generate cryptographic salt (16 chars)
- Create random split point in the key
- Add version and metadata
- Split key into server and client parts
-
Device Verification (iOS Only):
- Generate DeviceCheck token
- Get cryptographic validation from Apple
- Include token with every API request
-
Request Flow:
// Generate DeviceCheck token DCDevice.current.generateToken { token, error in guard let token = token else { print("DeviceCheck error: \(error?.localizedDescription ?? "unknown")") return } // Make API request with token and client part let url = URL(string: "https://api.proxed.ai/v1/structured-response")! var request = URLRequest(url: url) request.httpMethod = "POST" // Set required headers request.setValue("application/json", forHTTPHeaderField: "content-type") request.setValue(clientPart, forHTTPHeaderField: "x-ai-key") request.setValue(token.base64EncodedString(), forHTTPHeaderField: "x-device-token") request.setValue(projectId, forHTTPHeaderField: "x-project-id") // Request payload let payload = [...] request.httpBody = try? JSONSerialization.data(withJSONObject: payload) // Make the request URLSession.shared.dataTask(with: request) { data, response, error in if let error = error { print("Request error: \(error.localizedDescription)") return } guard let data = data, let json = try? JSONSerialization.jsonObject(with: data) else { print("Invalid response") return } // Handle the AI response print("Response: \(json)") }.resume() }
#Security Process
Here's the complete verification flow:
#Security Features
Our implementation includes multiple security layers:
-
Split-Key Protection:
- Server part stored securely in our infrastructure
- Client part stored on device with metadata
- Both parts required for key reconstruction
- Key reassembled only during request processing
-
Device Verification:
- Every request requires valid DeviceCheck token
- Tokens cryptographically signed by Apple
- Prevents non-iOS device access
- Automatic device validation
-
Audit & Control:
- Version tracking for key rotation
- Usage monitoring per device
- Suspicious activity detection
- Granular access control
#Implementation Example
Here's a real-world example of how the keys are split:
// Original API key
sk-abc123...xyz789
// After splitting
serverPart: "sk-abc1deadbeef" // Stored on Proxed
clientPart: "23...xyz789deadbeef.00000001000169df12345678"
^ ^ ^
version timestamp splitId
#Getting Started
To implement this security system:
- Create a Proxed account
- Configure your iOS app with DeviceCheck
- Generate and split your API keys
- Make API calls using our SDK
We handle all the complexity of:
- Key splitting and reassembly
- Device verification
- Usage tracking
- Security monitoring
#Future Enhancements
We're actively developing:
- Automated key rotation
- Enhanced usage analytics
- Advanced threat detection
- Multi-provider key management
- Additional platform support
Stay tuned for our next post about advanced key management strategies and best practices for secure key rotation.
