Secure Your AI API with One URL Change
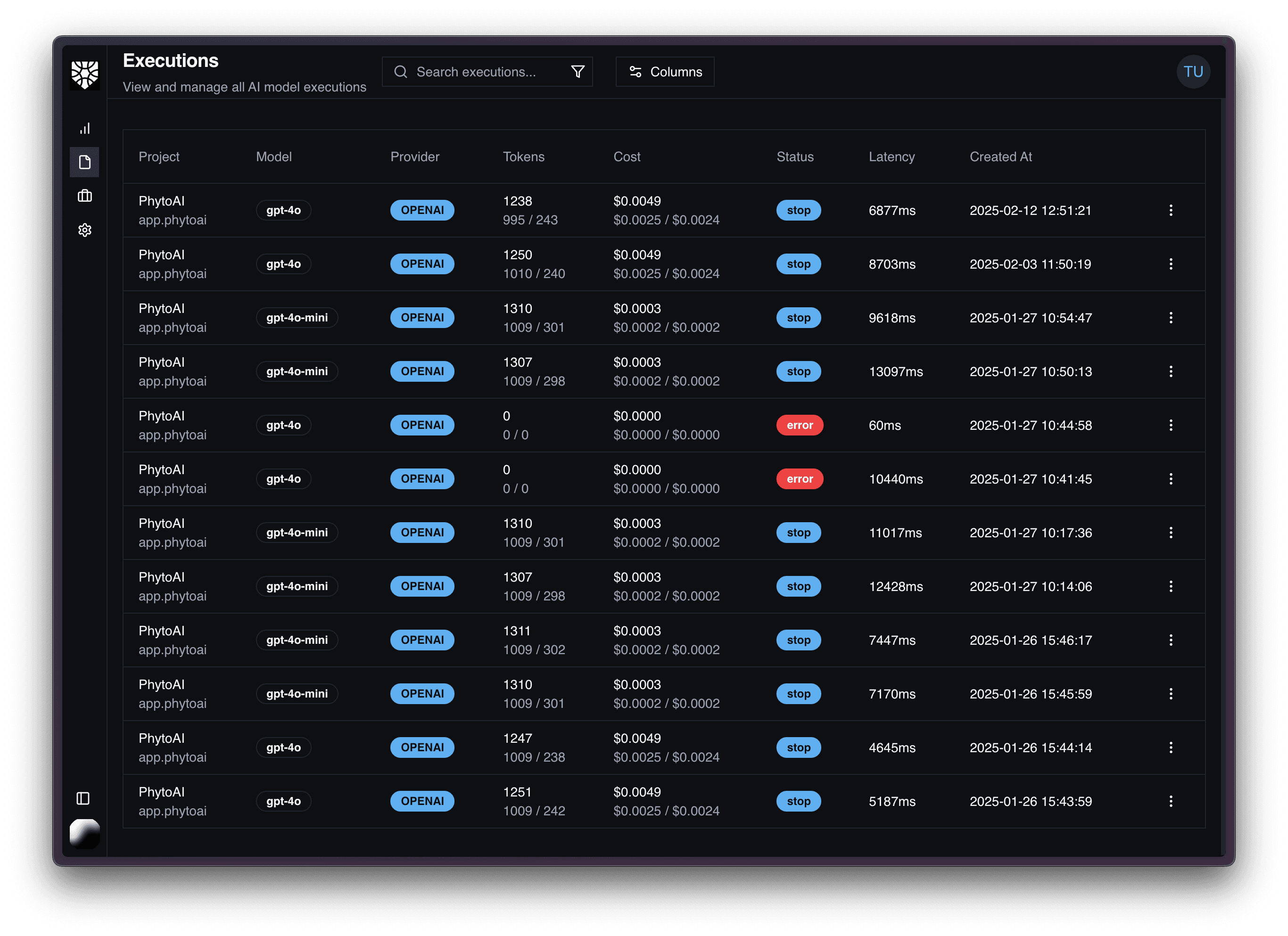
Simple Yet Powerful
Proxed.AI makes securing your AI integration as simple as changing a URL. No complex setup, no infrastructure changes—just point your API calls to our secure proxy and instantly gain enterprise-grade protection for your AI services. We handle device verification, API key management, and response validation while you focus on building great features.
Open Source Security
Security should be transparent. That's why Proxed.AI is open source, letting you verify our protection mechanisms and contribute to making AI integration safer for everyone. Our proxy service combines DeviceCheck verification, secure API key management, and structured response handling—all through a single URL change.
Implementation Example
import DeviceCheck
actor SimpleAPIIntegrator {
let apiKey = "<your-api-key>" // Partial API key (we don't store the full key)
let endpoint = "https://api.proxed.ai/v1/vision/<your-project-id>" // API endpoint
func sendImage(image: UIImage) async throws {
guard let imageData = image.jpegData(compressionQuality: 0.9) else {
fatalError("Image conversion failed")
}
let base64Image = imageData.base64EncodedString()
let token = await SimpleDeviceCheck.retrieveToken()
var request = URLRequest(url: URL(string: endpoint)!)
request.httpMethod = "POST"
request.setValue(apiKey, forHTTPHeaderField: "x-ai-key")
if let token = token {
request.setValue(token, forHTTPHeaderField: "x-device-token")
}
request.httpBody = try JSONEncoder().encode(["image": base64Image])
let (data, response) = try await URLSession.shared.data(for: request)
guard let httpResponse = response as? HTTPURLResponse,
(200...299).contains(httpResponse.statusCode) else {
fatalError("Failed to send image")
}
// Decode structured AI response which you build in the project settings from received data
let plantResponse = try JSONDecoder().decode(PlantResponse.self, from: data)
print("Plant Response:", plantResponse)
}
}
struct SimpleDeviceCheck {
static func retrieveToken() async -> String? {
guard DCDevice.current.isSupported else {
print("DeviceCheck not supported")
return nil
}
do {
let tokenData = try await DCDevice.current.generateToken()
return tokenData.base64EncodedString()
} catch {
print("Error generating token:", error)
return nil
}
}
}
struct PlantResponse: Decodable {
let scientificName: String
let commonNames: [String]
}
Key Benefits
- •Zero API keys in your client code
- •Automatic DeviceCheck verification
- •Real-time request monitoring and analytics
- •Rate limiting and cost control built-in