DeviceCheck Authentication
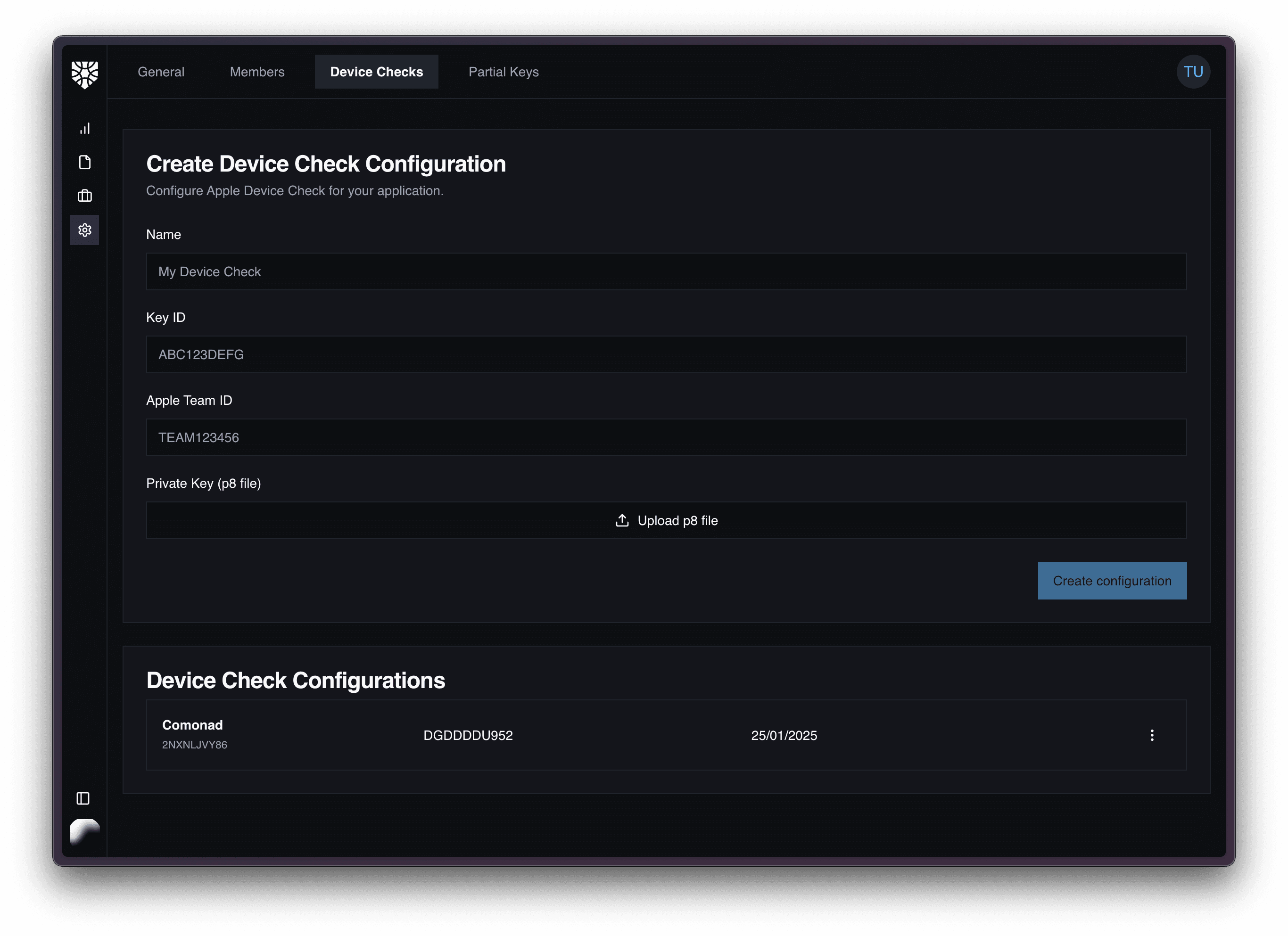
Hardware-Level Security
Proxed.AI integrates Apple's DeviceCheck to ensure only legitimate iOS devices can access your AI APIs. This powerful authentication system verifies device authenticity at the hardware level, blocking emulators, jailbroken devices, and automated requests that could compromise your API security.
Zero Configuration Required
Every request through Proxed.AI is automatically validated using Apple's secure DeviceCheck tokens. These cryptographic tokens prove that the request comes from a genuine Apple device, providing an additional layer of security beyond traditional API authentication methods. We handle all the DeviceCheck setup and validation—you just need to include the token in your requests.
Implementation Example
import DeviceCheck
struct DeviceCheckManager {
static func generateToken() async throws -> String {
guard DCDevice.current.isSupported else {
throw AuthError.deviceCheckNotSupported
}
let token = try await DCDevice.current.generateToken()
return token.base64EncodedString()
}
}
// Use in your API requests
let token = try await DeviceCheckManager.generateToken()
var request = URLRequest(url: URL(string: "https://api.proxed.ai/v1/chat/completions")!)
request.setValue(token, forHTTPHeaderField: "X-Device-Token")
// Proxed.ai automatically validates the token with Apple's servers
// and ensures the request comes from a legitimate device
let (data, response) = try await URLSession.shared.data(for: request)
// If device check fails, you'll receive a 403 Forbidden response
guard let httpResponse = response as? HTTPURLResponse,
httpResponse.statusCode == 200 else {
throw AuthError.invalidDevice
}
Key Benefits
- •Hardware-level device verification
- •Block emulators and jailbroken devices
- •Automatic token validation
- •No server-side setup needed